Recent Blogs
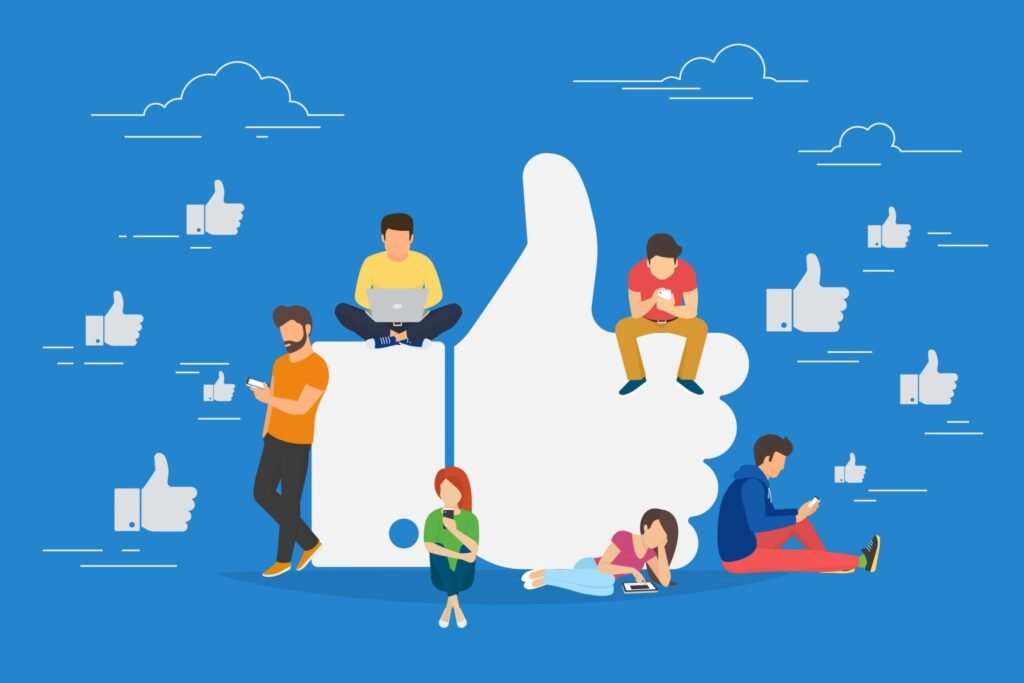
Step-by-Step Guide to Publishing a Private Vue 3 Components Library on npm
Vue 3 has become one of the most popular frameworks for building dynamic web applications, and sharing reusable components can significantly improve development efficiency. However, sometimes you may want to keep your component library private rather than making it publicly available on npm. In this guide, we will walk you through the step-by-step process of publishing a private Vue 3 components library to npm.
1. Set Up Your Vue 3 Component Library
Before publishing, you need a properly structured Vue 3 component library. If you don’t have one yet, start by creating a new project.
Key Steps:
Initialize a new npm package:
- mkdir vue3-private-library && cd vue3-private-library
npm init -y Install Vue 3 and necessary dependencies:
- npm install vue@next
Create a
src/
folder and add your Vue components.
2. Configure the Package for npm
To ensure your package is properly structured for npm, update the package.json
file.
Key Actions:
Add an entry point in
package.json
:- “main”: “dist/index.js”,
“module”: “dist/index.esm.js” Create an
index.js
file in thesrc/
folder to export components:import MyComponent from ‘./MyComponent.vue’;
export { MyComponent };
3. Build Your Library for Distribution
Since npm packages should not include raw .vue
files, you need to compile your component
Steps to Build
Install Vite or Rollup (popular build tools for Vue 3):
- npm install vite -D
Create a
vite.config.js
file:import { defineConfig } from ‘vite’;
import vue from ‘@vitejs/plugin-vue’;export default defineConfig({
plugins: [vue()],
build: {
lib: {
entry: ‘src/index.js’,
name: ‘MyLibrary’,
fileName: (format) => `my-library.${format}.js`
},
rollupOptions: {
external: [‘vue’],
output: {
globals: {
vue: ‘Vue’
}
}
}
}
});Run the build command:
- npm run build
4. Creating the Extension UI
Now, let’s modify App.vue to create a simple popup UI:
<template>
<div class=”popup”>
<h1>Vue 3 Extension</h1>
<button @click=”showMessage”>Click Me</button>
</div>
</template>
<script>
export default {
methods: {
showMessage() {
alert(“Hello from Vue 3 Extension!”);
}
}
}
</script>
<style>
.popup {
width: 200px;
padding: 10px;
text-align: center;
}
</style>
Explanation:
- Displays a simple h1 header.
- A button triggers an alert when clicked.
- Styled using basic CSS for a clean UI.
5. Publish the Private Package
After authentication, publish your package as private.
Steps:
Mark your package as private in
package.json
:- “private”: true,
“publishConfig”: {
“access”: “restricted”
} Publish the package:
- npm publish –access restricted
Conclusion
Publishing a private Vue 3 component library on npm allows you to share reusable components securely within your team. By following these steps—setting up the package, building for distribution, configuring authentication, and publishing—you can ensure seamless integration into your projects.
Need further guidance? Drop your questions in the comments or connect with us for expert support!